Generics
Line: |
Object Line |
|
Type: |
Walkthough |
You should have completed: |
Cast Away
|
This topic leads to: |
Music Shop
|
Summary
Sometimes it is useful to specify a type as one of the parameters to a method, this can increase code re-use and type safety as well as giving performance improvements. Generics are a way of doing this in the C# language for both individual methods and whole classes. You should already have encountered the concept whilst using Lists but the technique is more widely applicable.
In this exercise you will create a single method that can identify and retrieve a subset of an array of objects based on their type.
Task
- Create a console application.
- Create three new classes: Vehicle, Car and Bike.
- Car and Bike should both inherit from Vehicle.
- Edit the Program class to be the following.
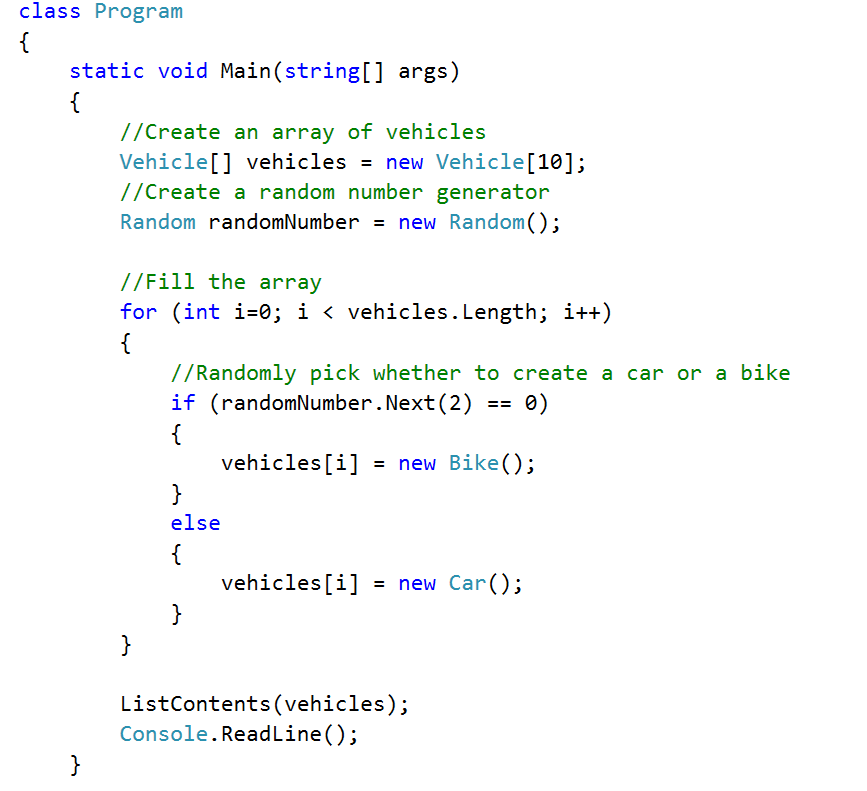
Here we have a class level variable to hold an array of 10 vehicles. In the Main() method we then randomly fill that array with Bike and Car objects. Finally we use a method to print out the contents of an array of vehicles.
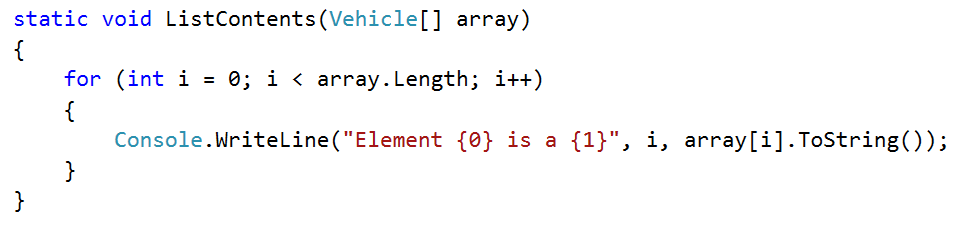
- We will now add the following code.
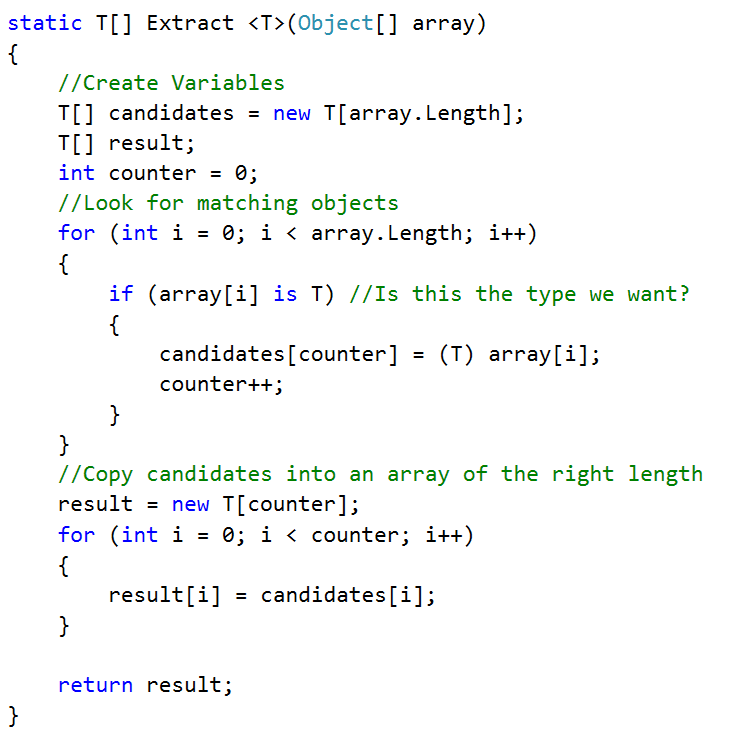
By using the generic syntax, we can leave the actual type of T until the method is called at runtime. Whatever type the user calls this method with, the code will work as though T was replaced with that type, it will also return an array of that type of object.
- In order to use this code to extract all the cars from our array, add the following code to the end of Main.
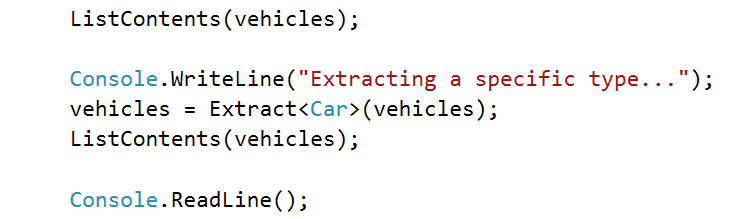
Questions
- How would you extract all the Bikes from the array?
- What would happen if you tried to extract all the Vehicles from the array?
- Can you now write a single function that will return the minimum of three numbers, and work for floats, doubles, integers and longs?
Notes
Further information can be found in the MSDN Reference Library.