Bank Account
Summary
This exercise demonstrates the combined use of static and non-static elements in a class, as well as demonstrating the value and use of encapsulation to improve the integrity and maintainability of code.
Task
- Create a console application.
- Add a new class, called BankAccount to the project (You can use the Project>>Add Class... menu)
- Type in the following code into your new class
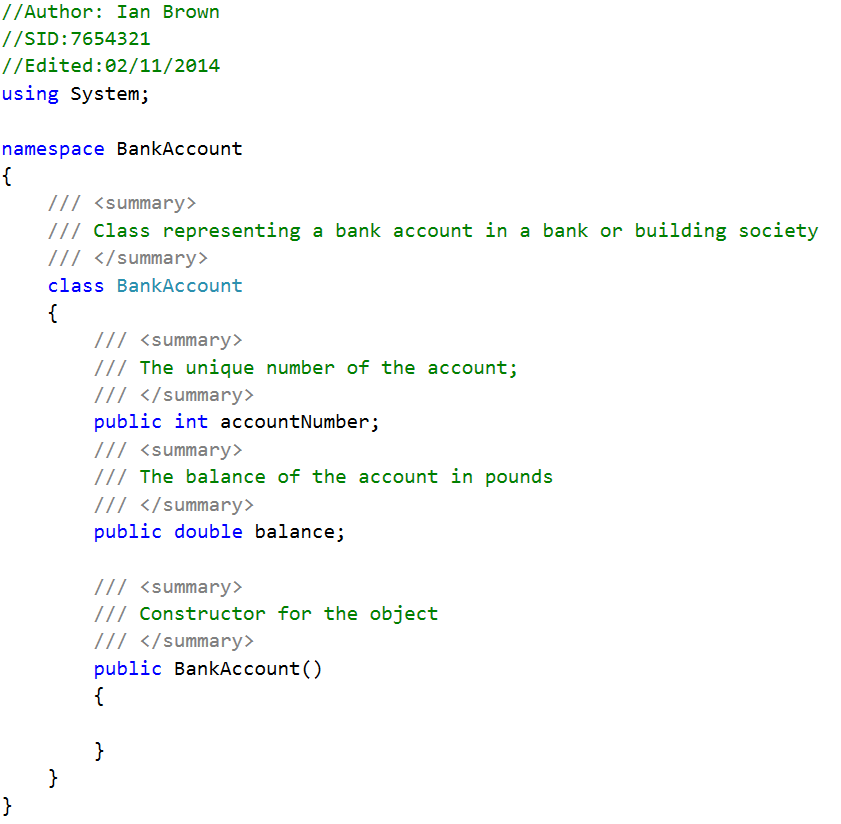
- Then edit your program class to use this class as follows
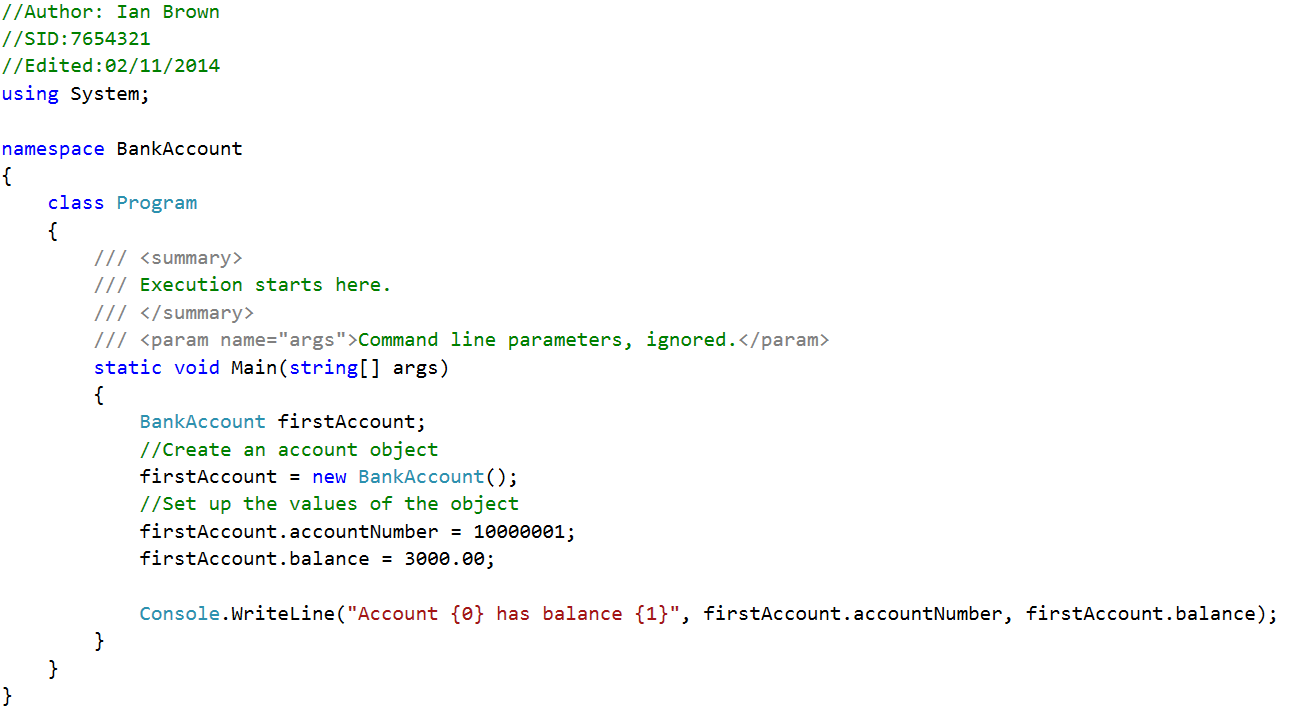
We now have a class, with a default constructor, and simple 'test harness' in the code in main which creates an object of that class and uses it. This class does not follow the principle of encapsulation at all, however, and is therefore quite poorly designed right now.
- Add a method 'Withdraw' to the BankAccount class as follows.
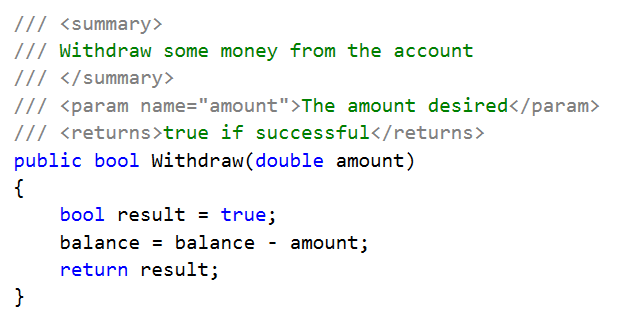
- Now add code to the Main method to withdraw £5,000 from the account and report whether this is successful.
- Run the code and note that even though the account has only £3k in it, we can withdraw more.
- Edit the Withdraw method to check that there is enough money in the account to make the withdrawal, and if not, change the return value to false and do not decrease the account balance.
- Run the code again. This time the withdrawal should not be successful.
- Is there a way you could still reduce the account balance by £5,000 from Main and how?
- We can fix this by 'encapsulating' the balance information inside the class, and allowing only controlled access to it through members on the interface of our class (of which Withdraw will be one). From here, this is a 4-step process (though in future you would design your classes to be encapsulated from the beginning.
- Make the balance variable private, and accessible through a Property: Right-click on the definition of balance and select Refactor>>Encapsulate Field.
- Now we need to make the property read-only: Delete the set part of the property definition.
- Finally, since we can no longer set the balance of the account directly, we need a 'Deposit' method, so write one. Note that this may be called several times and shoud therefore add any money deposited to the existing balance.
- Edit your Main method to work with these changes.
- With the account balance properly encapsulated, there should now be no way from Main to decrease it below 0. The accountNumber however is still open to uncontrolled editing. Ideally, account numbers should be unique, and there is currently no way of enforcing that. The following steps will fix this...
- Make the accountNumber a read-only property, as we did with balance.
- Add a static class-level variable called nextAccountNumber to the BankAccount class, initialise it to 10000000. This variable belongs to the class and is shared across all objects of that class. It will have the same value wherever in code it is accessed from.
- In the constructor of BankAccount, set the private accountNumber variable to the value of nextAccountNumber, and then increment nextAccountNumber. This ensures that every time you create an account it automatically has a unique account number.
- Edit your Main to work with these changes, and then to create a second bank account to test that it has a different account number.
Questions
- How can you add an overdraft to this bank account class? Fully test your solution.
- Can you add a method to the bank account to determine if this is a good client of the bank (e.g. if they have more than £10,000 in their account).
- Add the ability to set an initial balance and the customer's name through the constructor. Hint: you may need to add more variables or parameters.