Virtual Student
Summary
Object oriented programming works through expert objects sending messages to each other, requesting data and services from each other. Those objects which contain the data also contain the expertise, in the form of methods, for manipulating that data. This example will calculate a grade for a virtual student by combining the grades for multiple modules, the modules will calculate their grades by combining the marks for multiple assessments. The expert knowledge is contained at the relevant level of the program.
For mathematical simplicity you are advised to treat all marks and weightings as 'normalised' values between 0 and 1. Therefore 70% should be expressed as 0.7 etc.
Task
- Create a console application.
- In the Program class, write the following code. It won't compile yet as we'll need to create the functionality later in the exercise.
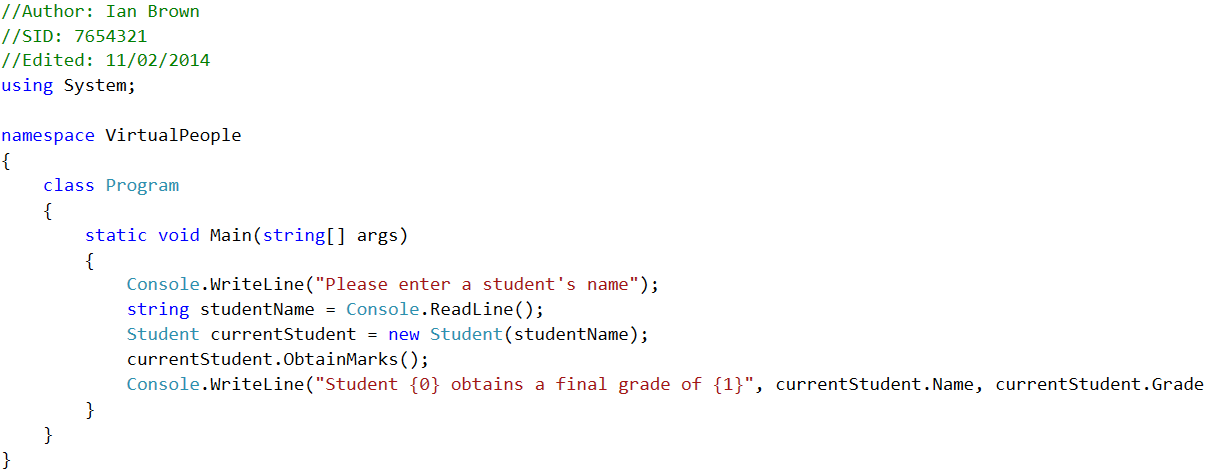
This program will eventually ask the user for the marks for a given student, then calculate their final grade.
- Create a Student class. Give it a properly encapsulated Name attribute and a constructor that takes a string and assigns that value to the name.
- Create an ObtainMarks method on the Student class that has no return value (void).
- Create a read-only Grade property which is of type float, and for now simply return 0 as that value.
- Test that the code now compiles and runs.
- Add two new classes to the project, Module and Assignment
- Assignment needs the following read-only attributes, and a constructor that allows all to be set.
- Marks, float //The marks given for this assignment, 0-1
- Pass, bool //Whether the marks are >= 0.30
- Weighting, float //How much of the module grade is from this assignment.
- Module needs the following read-only attributes, and a constructor that allows all to be set.
- Name, string //the module name
- NumberOfAssignments, int //How many assignments for this module (1-4)
- Credits, int //How many credits is this module worth. 15, 30, 45 or 60
- Add a private array of Modules as a class level variable in the Student class
- Add a private array of Assignments as a class level variable in the Module class
- In the Student class constructor add the following functionality
- initialise the array of modules to size 4 and create one module object of each credit size and store them in the array - you can make up the module names, and pick how many assignments each has.
- In the Module class constructor add the following functionality
- initialise the array of assignments to NumberOfAssignments and create one Assignment object and store it in each element of the array - give all the assignments equal weighting.
- In a fully functional program marks would be obtained from a database, but for now we must cheat a little and ask the user (Ideally avoid using Console commands within objects intended for data manipulation as it makes them much less reusable). Write a public ObtainMarks() method on the Assignment class. This method must ask the user for the marks for this assignment, and then store those in the marks attribute.
- Now write an ObtainMarks() method on the module class which iterates through all the assignments of that module and asks them to use their ObtainMarks() methods.
- Now edit the ObtainMarks() method on the student, to ask each Module object in turn to obtain marks. See how this pattern of 'passing the buck' works.
- Run the program and get it asking for the correct set of marks. Once it is working, we can be sure of getting data to manipulate.
- Add a GetGrade() method to the assignment class that returns a float. Calculate the return value as follows:
if the Pass attribute is true, return the marks
otherwise, return 0.4 (We will assume that if a student fails an assignment on the first try, they will succeed at a resit).
- Add a GetGrade() method to the module class that returns a float. Calculate the return value as the sum over all assignments of the (weighting*grade).
- Finally edit the Grade property of the student to return a value calculated as the (sum over all modules of (module grades * credits) ) / total credits for all modules. Also known as the credit-weighted mean. Note here that you can calculate the value for a property on the fly, rather than having to return the value of a variable.
- Run and test this program. Note how the Module class does not need to know how each assignment calculates its grade, and the Student class does not need to know how various assignment results make up the Module grade.
Questions
- This example is not fully representative of the University's marking and calculations. How can you adjust the Student.Grade calculation to reflect Anglia Ruskin University's grading algorithm described in the Academic Regulations section x.xxx? Hint: you may need to add a 'level' attribute to the Module class.
- How can you adjust the modules in the program to reflect your own course structure?